while loop in C
Loops programming construct is used to execute one or more instructions repeatedly until some condition is satisfied.
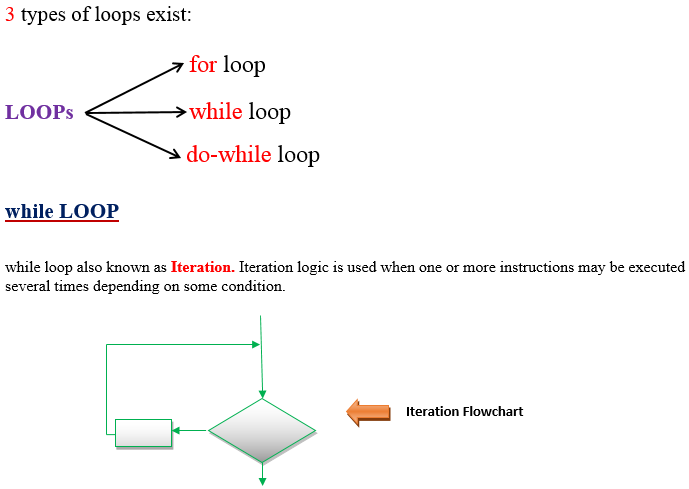
ITERATION
oIteration comes from the word “reiterate”, which means to repeat
oIteration is a looping construct
oIteration is a combination of decision and sequence and can repeat steps
oIteration can be thought of as “while something is true, do this, otherwise stop”
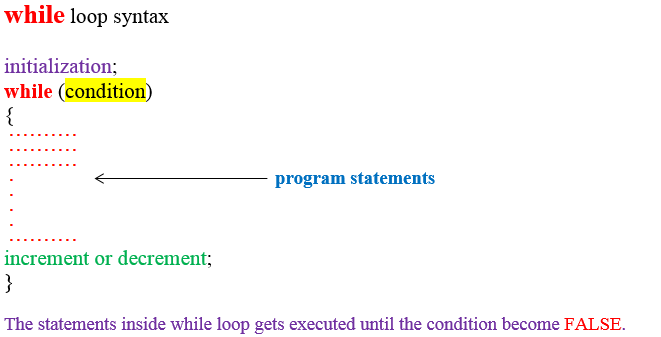
For loop also known as iterative statement. To iterate means to repeat. If we want to repeatexecution of some action or statements several times, we use LOOPs.
Practice Programs
(i)
#include<iostream>
#include<cstdlib>
using namespace std;
void main()
{
system("color fc");
int x;
x = 0;
while(x<5)
{
printf("Stephen Hawking \n");
x++;
}
}
(ii)
#include<iostream>
using namespace std;
void main()
{
int x, y;
x=1;
while(y = 5)
{
printf("Stephen Hawking \n");
x++;
}
}
(iii)
#include<iostream>
using namespace std;
void main()
{
int x, y = 10;
x=2;
while(y == 10)
{
printf("Stephen Hawking \n");
x++;
}
}
(iv)
#include<iostream>
using namespace std;
void main()
{
int i, x, y;
x = 2;
y = 3;
i=2;
while(x+y)
{
printf("Stephen Hawking \n");
i++;
}
}
(v)
#include<iostream>
using namespace std;
void main()
{
int i, x, y;
x = 2;
y = 3;
i=2;
while((x+y)>0)
{
printf("Stephen Hawking \n");
i++;
}
}
(vi)
#include<iostream>
using namespace std;
void main()
{
int i, x, y;
x = 2;
y = 3;
i=2;
while((x+y)<0)
{
printf("Stephen Hawking \n");
i++;
}
}
(vii)
#include<iostream>
using namespace std;
void main()
{
int i;
i=2;
while(i<=5)
{
printf("Stephen Hawking \n");
printf("Galaxy \n");
i++;
}
}
(viii)
#include<iostream>
using namespace std;
void main()
{
int i, x, y;
for(i = 2; i<=5; i++)
printf("Stephen Hawking \n");
printf("Cosmologist \n");
printf("Galaxy \n");
}
(ix)
#include<iostream>
using namespace std;
int main()
{
int i, x, y;
i=2;
while(i<=5)
{
printf("Stephen Hawking \n");
printf("Cosmologist \n");
printf("Galaxy \n");
i++;
}
}
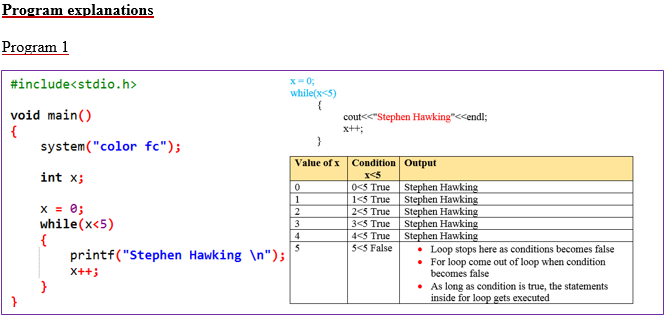
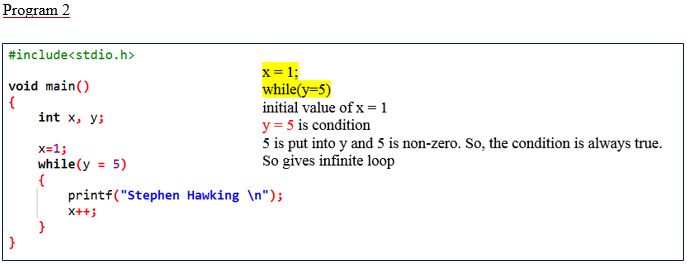
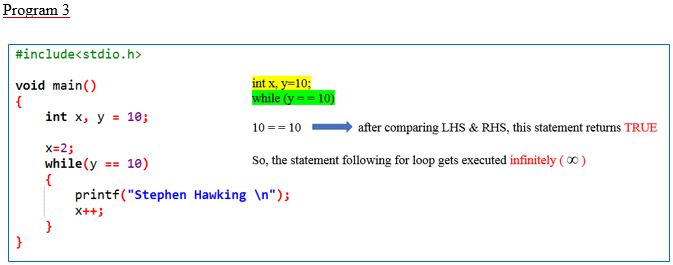
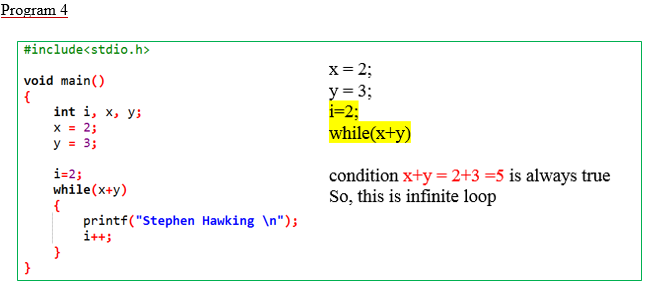
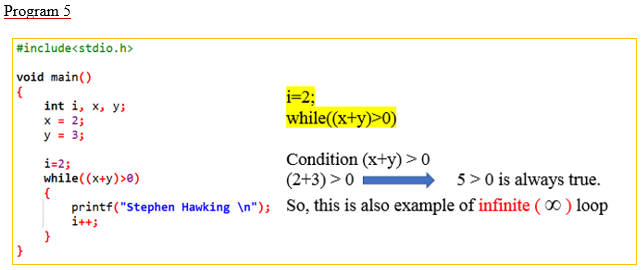
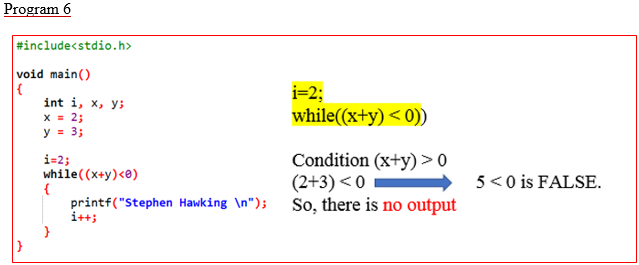
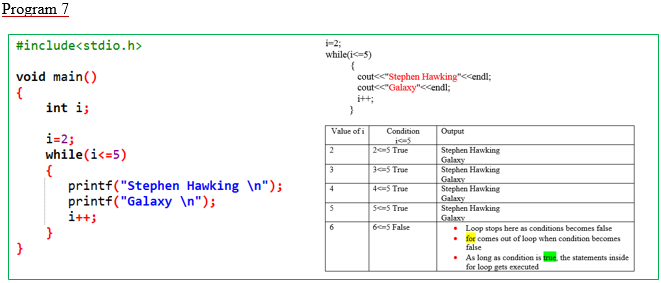