Simple if – else statement in C
Simple if – else Syntax
The form of an if statement is as follows:
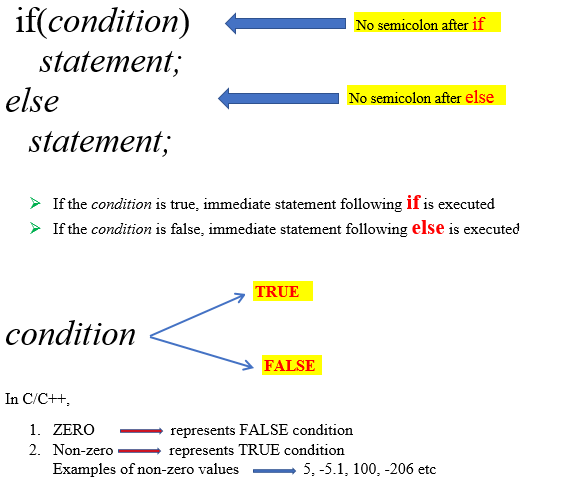
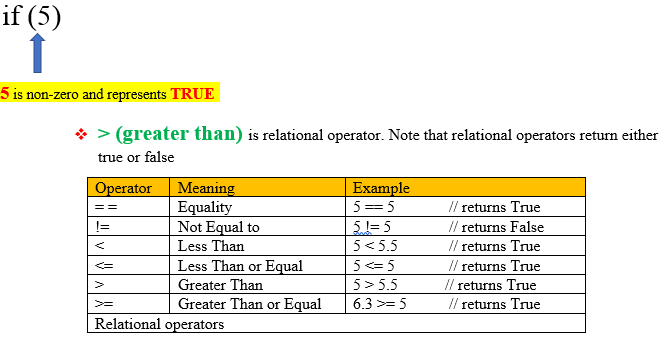
Note that every operator in C++ must return some value. For example, + operator returns sum of two numbers, * operator return multiplication of two numbers etc.
Practice Programs
(i)
#include<stdio.h>
void main()
{
int x = 5;
if(x)
printf("Stephen Hawking");
else
printf("Albert Einstein");
}
(ii)
#include<stdio.h>
void main()
{
int x = 5;
if(x>10)
printf("Stephen Hawking");
else
printf("Albert Einstein");
}
(iii)
#include<stdio.h>
void main()
{
int x = 5;
if(x == 10)
printf("Stephen Hawking");
else
printf("Albert Einstein");
}
(iv)
#include<stdio.h>
void main()
{
int x = 5, y = 10;
if(x+y)
printf("Stephen Hawking");
else
printf("Albert Einstein");
}
(v)
#include<stdio.h>
void main()
{
int x = 5, y = 10;
if( (x+y)>30 )
printf("Stephen Hawking");
else
printf("Albert Einstein");
}
(vi)
#include<stdio.h>
void main()
{
int x = 5, y = 10;
if( (x+y)>30 )
{
printf("Stephen Hawking\n");
printf("Cosmology");
}
else
{
printf("Albert Einstein\n");
printf("Physics");
}
}
(vii)
#include<stdio.h>
void main()
{
int x = 5, y = 10;
if( (x+y)>30 )
printf("Stephen Hawking\n");
printf("Cosmology");
else
printf("Albert Einstein\n");
printf("Physics");
}
(viii) Testing for Leap year
#include<stdio.h>
void main()
{
int year;
printf("enter year \n");
scanf("%d", &year);
if((year%400==0)||((year%4==0)&&(year%100!=0)))
printf("given year is leap year \n");
else
printf("not leap year \n");
}
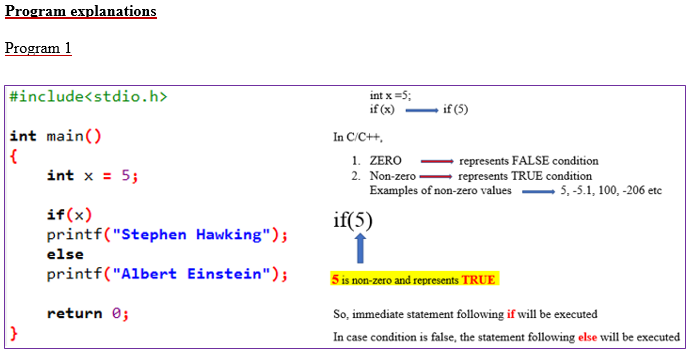
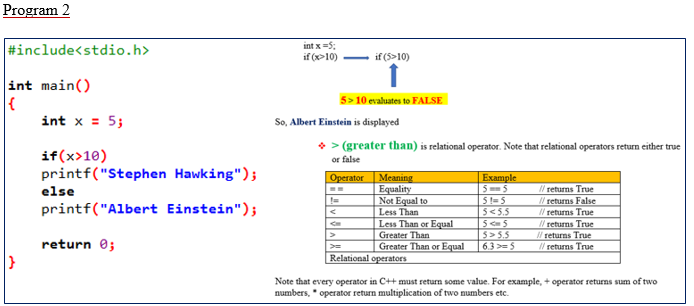
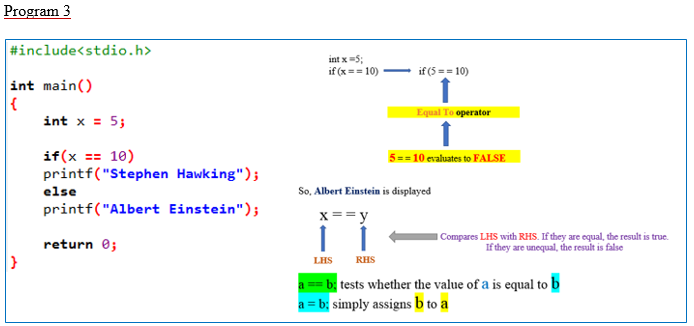
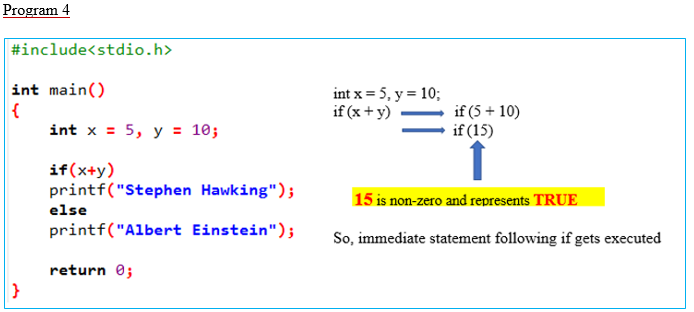
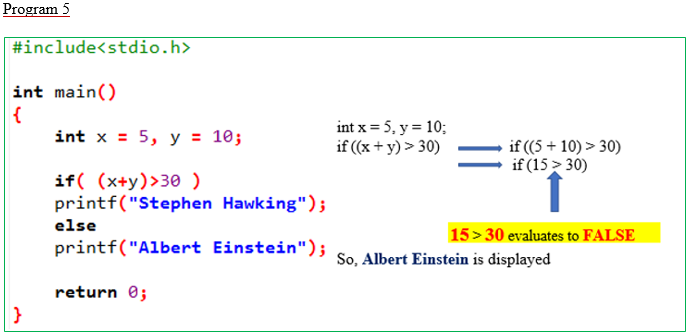
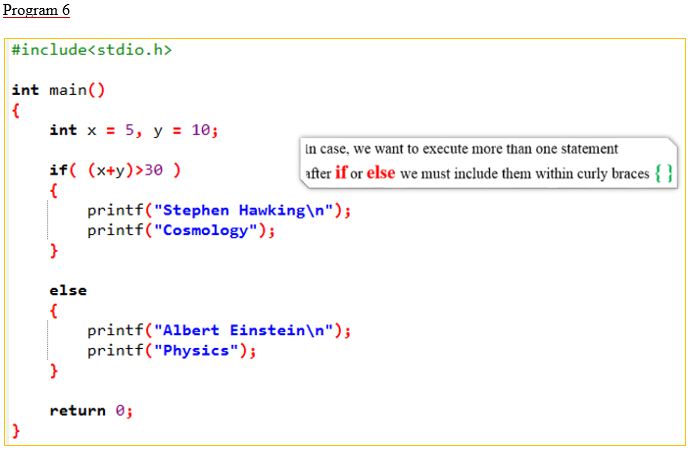
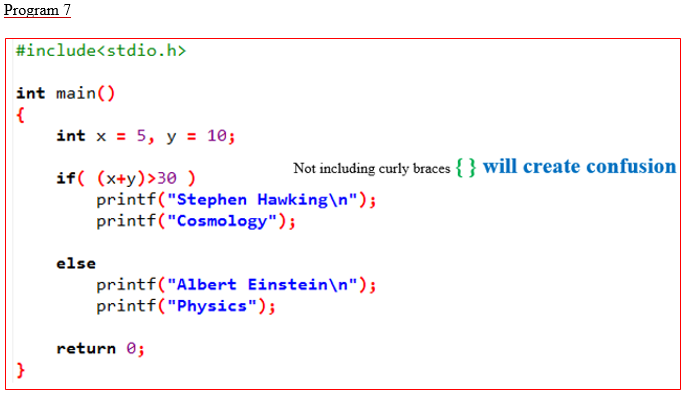
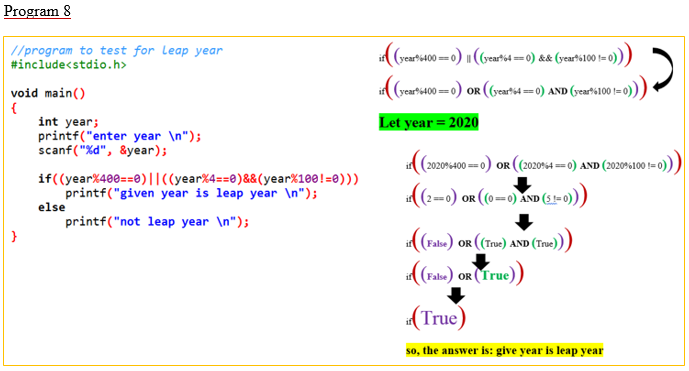